本文首发于 2014-01-10 19:48:48
1. getopt
该函数用来解析命令行参数。
1.1. 函数定义
1 | int getopt(int argc, char * const argv[], const char *optstring); |
前两个参数设为 main 函数的两个参数。
optstring 设为由该命令要处理的各个选项组成的字符串。选项后面带有冒号’:’时,该选项是一个带参数的选项。
举例:
make -f filename -n
-f 是一个带参数的选项,-n 是一个没有参数的选项。可以像下面这样调用
函数getopt
来解析上面的例子。
1 | c = getopt(argc, argv, "f:n"); |
此函数的返回值即为当前找到的命令选项,全部选项都找到时的返回值为-1。
通常一个命令有多个选项,为了取得所有选项,需要循环调用此函数,直到返回值为-1。
要使用此函数,还有几个全局变量必须要了解:
1 | extern char *optarg; |
1.2. 实例
1 |
|
2. getopt_long
这是支持长命令选项的函数,长选项以’–’开头。
2.1. 函数定义
1 | int getopt_long(int argc, char * const argv[], |
前三个参数与函数 getopt 的参数是一样的。
只支持长选项时,参数 optstring 设置为 NULL 或者空字符串””。
第四个参数是一个构造体 struct option 的数组。此构造体定义在头文件 getopt.h 中。此数组的最后一个须将成员都置为 0。
1 | struct option { |
构造体各个成员的解释如下:
- name : 长选项的名字。
- has_arg : no_argument 或 0 表示此选项不带参数,required_argument 或 1 表示此选项带参数,optional_argument 或 2 表示是一个可选选项。
- flag : 设置为 NULL 时,getopt_long()返回 val,设置为 NULL 以外时,>getopt_long()返回 0,且将*flag 设为 val。
- val : 返回值或者*flag 的设定值。有些命令既支持长选项也支持短选项,可以通过设定此值为短选项实现。
第五个参数是一个输出参数,函数 getopt_long()返回时,longindex 的值是 struct option 数组的索引。
关于返回值有以下几种情况:
- 识别为短选项时,返回值为该短选项。
- 识别为长选项时,如果 flag 是 NULL 的情况下,返回 val,如果 flag 非 NULL 的情况下,返回 0。
- 所有选项解析结束时返回-1。
- 存在不能识别的选项或者带参数选项的参数不存在时返回’?’ 。
2.2. 实例
1 |
|
欢迎关注我的微信公众号【数据库内核】:分享主流开源数据库和存储引擎相关技术。
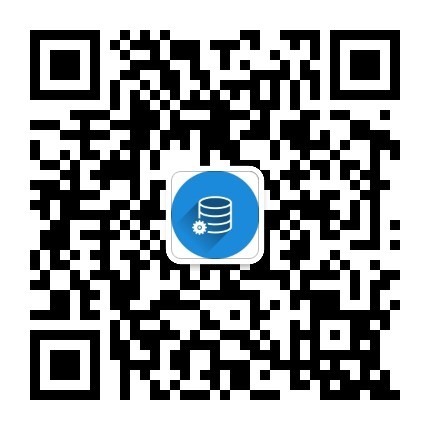
标题 | 网址 |
---|---|
GitHub | https://dbkernel.github.io |
知乎 | https://www.zhihu.com/people/dbkernel/posts |
思否(SegmentFault) | https://segmentfault.com/u/dbkernel |
掘金 | https://juejin.im/user/5e9d3ed251882538083fed1f/posts |
CSDN | https://blog.csdn.net/dbkernel |
博客园(cnblogs) | https://www.cnblogs.com/dbkernel |